Debugging is an essential part of software development, and mastering it can dramatically improve both the speed and quality of your work. While coding is often creative and logical, debugging can feel more like detective work—tracing through complex systems and scenarios to find the hidden cause of an issue.
In this guide, we’ll dive deep into 10 essential debugging techniques that will help you quickly identify and resolve bugs in your code, ensuring smoother development and more reliable software.
1. Reproducing the Bug
Why It’s Crucial:
Before you can fix a bug, you must be able to reproduce it consistently. Without a reproducible scenario, it’s difficult to track down the exact conditions under which the bug occurs and to verify that your fix actually resolves the issue.
Steps to Reproduce:
- Isolate the Problem: Narrow down the conditions under which the bug appears. Does it happen with specific inputs, in certain environments (OS, browser, device), or after certain actions?
- Recreate the Environment: Try to mirror the exact environment where the bug was first encountered. This includes using the same operating system, browser, database, or device. Sometimes subtle differences in versions or configurations can introduce bugs.
- Use Logs and Monitoring: Logging can help track down when and why the bug occurs. For example, logging variable values or state changes right before the crash or error can highlight the issue.
- Break Down Complex Interactions: If the bug is elusive, start simplifying. Turn off certain features or components one by one to see which part of the system is causing the problem.
Tools:
- Virtual Machines and Containers: Tools like Docker allow you to recreate specific environments for testing bugs.
- Logging Libraries: Tools such as
Winston
in Node.js,Log4j
in Java, or nativeprint()
statements are useful for tracking down issues.
Example:
Imagine a bug that only occurs when a user logs in from a mobile device. Reproducing the bug may involve:
- Testing the login flow in a mobile emulator or physical device.
- Comparing network traffic between mobile and desktop logins using tools like Chrome DevTools.
- Verifying that the bug appears consistently when following the same steps.
2. Using Print Statements (and Logging)
Why It Works:
Print statements are a tried-and-true debugging technique, often the first tool developers reach for. They allow you to quickly inspect the flow of the program and the values of variables without stopping execution. Logging is essentially the professional version of print statements, offering more features such as log levels and persistent records.
How to Use Print Statements Effectively:
- Trace Program Flow: Add print statements at key locations in your code to ensure that execution is happening as expected. For example, if a function isn’t working correctly, printing at the start and end of the function can confirm if it’s being called at all.
- Check Variable Values: Print variables at crucial points in your code to see if they hold the expected values. This can help identify logic errors, incorrect data passing, or failed condition checks.
Logging: A Step Up from Print Statements
- Log Levels: Logging libraries typically support different levels (DEBUG, INFO, WARN, ERROR), allowing you to easily filter the logs based on importance.
- Persistent Logs: Logs are often stored in files or external systems, making it easier to track long-running applications or distributed systems where print statements might not be sufficient.
Tools:
- Log4j (Java), Winston (Node.js), Pino (Node.js), Python’s
logging
module
3. Using Debuggers
Why Debuggers Are Essential:
Debuggers allow for a more interactive approach to debugging, letting you pause execution, inspect variables, and step through the code one line at a time. This is invaluable for tracking down hard-to-find bugs, especially in asynchronous or multi-threaded code.
Key Features of Debuggers:
- Breakpoints: Set a breakpoint to pause the program at a specific line. This lets you inspect the state of the program, including variable values and the call stack, at that precise moment.
- Stepping: You can “step over” to execute the next line or “step into” to dive deeper into a function call.
- Watch Variables: You can monitor specific variables or expressions as the program runs, ensuring they hold the expected values throughout execution.
Debugger Example:
Imagine you’re working on a web app and notice that form data isn’t being saved correctly. By using Chrome Developer Tools, you can:
- Set a breakpoint at the form submission function.
- Step through each line of code to see where the data is being processed incorrectly.
- Inspect network requests to ensure the data is being sent correctly to the server.
Popular Debugging Tools:
- Chrome DevTools (JavaScript debugging in browsers)
- PyCharm, VSCode Debugger (for Python)
- Xcode Debugger (iOS development)
- GDB (GNU Debugger) for C/C++
4. Rubber Duck Debugging
What is Rubber Duck Debugging?
Named after a method where developers would explain their code to an inanimate rubber duck, this technique is based on the simple act of explaining your problem out loud. The process of articulating the problem often leads to a better understanding and can reveal mistakes.
Why It Works:
- Verbalizing Your Thought Process: By explaining your code, you force yourself to think about it from a higher level, often revealing logical flaws or overlooked details.
- Breaks Tunnel Vision: When you’re deeply focused on solving a problem, it’s easy to miss simple errors. Explaining the issue forces you to think differently.
How to Do It:
- Talk Through Your Code: Pretend that you’re explaining the code or issue to a beginner. This helps you rethink assumptions or hidden complexities.
- Ask Questions: Even if you’re alone, ask questions as if you’re in a conversation: “What is this variable supposed to be here? Why am I passing this argument?”
5. Binary Search in Code
Why It’s Effective:
When you don’t know exactly where the problem is in a large codebase, stepping through every line of code can be time-consuming. Instead, binary search divides the code in half repeatedly, allowing you to quickly narrow down the bug’s location.
How It Works:
- Divide the Code in Halves: Disable or skip half of the code to see if the bug still occurs.
- Continue Dividing: If the bug persists, the issue is in the active half. If the bug disappears, it’s in the disabled half. Continue this process until you isolate the exact line or block causing the bug.
Example:
If you have a bug in a 1,000-line function, you could start by commenting out the first 500 lines. If the bug persists, it’s in the latter 500 lines. Keep halving the code (e.g., lines 500-750, 750-875, etc.) until you find the exact problem.
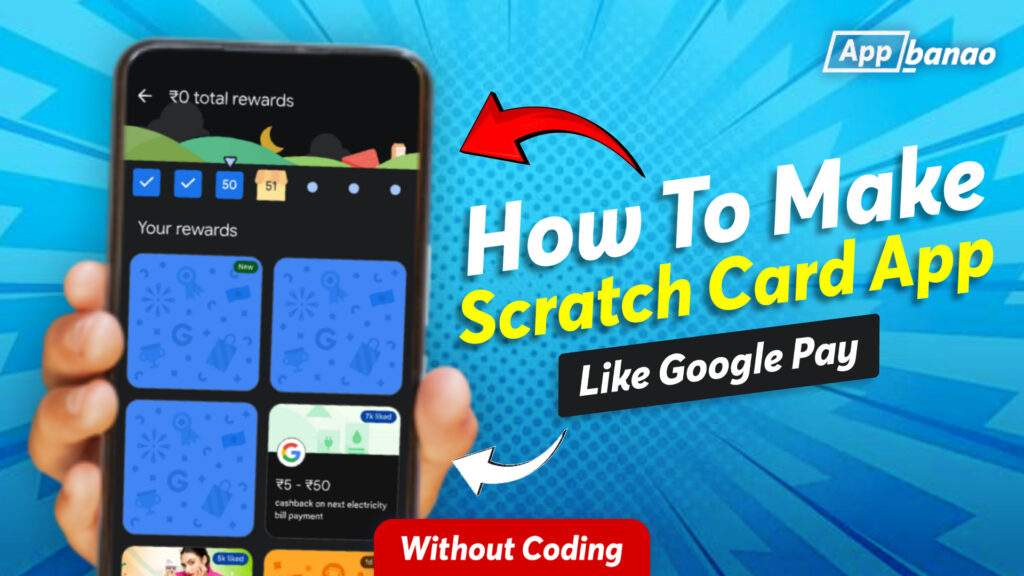
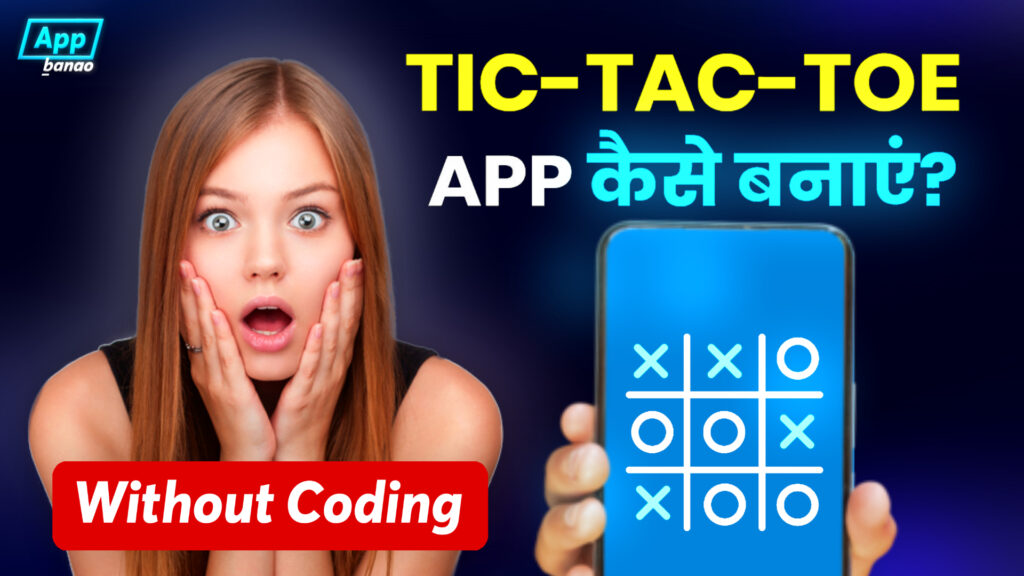
6. Analyzing Stack Traces
Why Stack Traces Are Valuable:
When a program crashes or throws an error, a stack trace provides a snapshot of the function call hierarchy at the point of failure. This can help you track down where the bug originated and why it happened.
How to Read a Stack Trace:
- Identify the Error: The first few lines of the stack trace usually contain the actual error message, such as a
NullPointerException
,SyntaxError
, orTypeError
. This tells you what kind of issue you’re dealing with. - Trace the Function Calls: The stack trace lists the chain of function calls that led to the error. By following these calls, you can understand the flow of execution that caused the bug.
Example:
If you encounter a NullPointerException
in Java, the stack trace will show the exact line of code where the null value was accessed, along with the sequence of function calls that led to it. From there, you can trace back to see why the value was null in the first place.
7. Version Control for Debugging (Git Bisect)
Why It’s Useful:
When you’re working in a large codebase or collaborating with a team, it can be difficult to pinpoint when and where a bug was introduced. Git Bisect uses a binary search algorithm to identify the commit that caused the issue, saving you from manually sifting through hundreds of changes.
How to Use Git Bisect:
- Start Git Bisect: Begin the process by marking the current (buggy) commit as bad and a previous (working) commit as good:sql
- Test Midpoints: Git will automatically check out a commit in the middle of the two. Test if the bug still exists. If yes, mark it as
bad
; if no, mark it asgood
. - Continue Bisecting: Git will keep narrowing down the range of commits until it identifies the exact commit that introduced the bug.
Pro Tip:
When using Git Bisect, try to automate the testing process. You can create a test script that returns 0
for a good commit and 1
for a bad commit, allowing Git to automatically run the bisect process.
8. Checking for Off-by-One Errors (OBOEs)
Why Off-by-One Errors are Common:
Off-by-one errors occur when a loop or range includes one iteration too many or too few, usually caused by incorrect boundary conditions. These errors are subtle but can cause significant issues in programs that involve indexing, such as when working with arrays or lists.
How to Avoid Off-by-One Errors:
- Carefully Check Loop Conditions: Ensure that your loops are correctly bound. For example, make sure you understand the difference between
<=
and<
in loops.
- Use Built-in Iterators: Languages like Python provide iterators (e.g.,
for ... in
loops) that manage boundaries for you, reducing the chance of off-by-one errors.
9. Peer Debugging (Pair Programming)
Why It Works:
Having a second set of eyes on your code can reveal issues that you might have overlooked. Peer debugging or pair programming helps you share insights and approaches, leading to faster bug detection and resolution.
How to Do Peer Debugging:
- Explain the Problem: Start by explaining the problem to your peer. In doing so, you may clarify the issue for yourself.
- Work Together: Take turns navigating the code. Your peer might notice things you missed, like logic errors, unused variables, or missing edge cases.
- Ask for Fresh Insights: Sometimes, another developer can offer a fresh perspective on the problem, especially if you’ve been too focused on one specific area.
Pro Tip:
Even if your peer doesn’t have detailed knowledge of the project, explaining the code can help clarify your understanding and spark new ideas for debugging.
10. Testing Edge Cases
Why Testing Edge Cases Matters:
Bugs often surface in situations that aren’t part of the “normal” usage path—these are called edge cases. They might involve empty inputs, extremely large datasets, or unusual user behaviors.
How to Test Edge Cases:
- Identify Edge Cases: Think about the unusual or extreme scenarios that your code might encounter. For example, what happens if a user submits an empty form? What if an array has a single element, or 10 million elements?
- Write Unit Tests for Edge Cases: When creating test cases, ensure that you include tests for edge conditions. For example, if you’re writing a function to calculate the average of a list of numbers, test what happens with an empty list or a list with only one number.
- Simulate Real-World Extremes: Sometimes edge cases relate to the environment. For example, how does your app handle low-memory conditions, or high-latency network environments? Use tools to simulate these scenarios.
Conclusion
Debugging is an essential skill that requires both logical problem-solving and creativity. By mastering these techniques—ranging from basic print statements to advanced tools like debuggers and Git Bisect—you’ll be able to tackle a wide range of bugs, reduce downtime, and write more reliable, efficient code. Whether you’re working on a small personal project or maintaining a large codebase, the time spent honing your debugging skills will pay off in the long run.
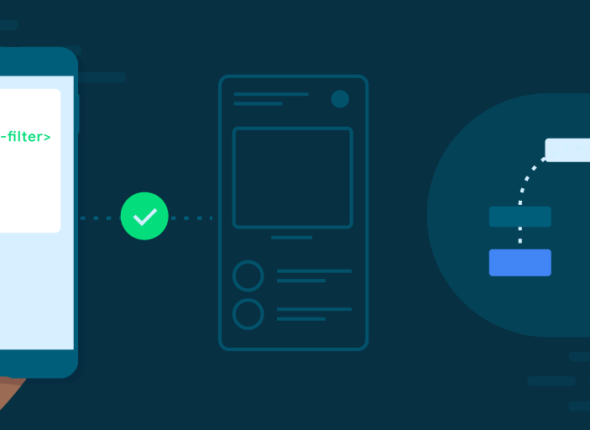
Tips for Reducing App Load Time and Memory Usage
In today’s fast-paced digital world, users expect mobile apps to perform flawlessly, load quickly, and...
- October 17, 2024
- Com 0