APIs (Application Programming Interfaces) play a critical role in modern application development, enabling communication between client applications and backend services. Whether it’s a mobile app, web app, or IoT device, APIs are often the backbone that allows applications to retrieve and send data. However, with this increased reliance on APIs comes an inherent risk: unsecured API endpoints can become an entry point for attackers to steal data, execute malicious operations, or compromise the entire system.
In this blog, we will explore why securing API endpoints is crucial, common vulnerabilities, and best practices every developer should follow to ensure their APIs remain secure.
Why Securing API Endpoints Is Crucial
API security is essential for the following reasons:
1. Protection of Sensitive Data
APIs are often the gateways to sensitive data, including personal information, financial records, and proprietary business data. If attackers gain access to unsecured APIs, they can steal, alter, or delete this data, leading to severe consequences like identity theft, data breaches, and financial loss.
2. Preventing Unauthorized Access
A secure API ensures that only authorized users and systems can interact with it. Without proper security measures, unauthorized users can potentially access confidential data or execute harmful actions.
3. Maintaining Trust
API security is critical for maintaining the trust of users and partners. Data breaches due to poorly secured APIs can severely damage a company’s reputation and lead to loss of business.
4. Regulatory Compliance
In many industries, securing APIs is necessary to comply with regulatory standards like GDPR, HIPAA, or PCI-DSS. Failure to secure APIs can result not only in data breaches but also in hefty fines and legal penalties.
Common API Vulnerabilities
Before we delve into best practices, let’s look at some common vulnerabilities that can arise if API endpoints aren’t secured properly:
1. Lack of Authentication and Authorization
APIs that do not require proper authentication or authorization are highly vulnerable. Without these measures, anyone with access to the API endpoint could perform actions or retrieve data meant for authorized users only.
2. Injection Attacks
APIs that accept user input without proper validation are susceptible to injection attacks, such as SQL injection or command injection. Attackers can manipulate input to execute harmful SQL queries or commands on the backend server.
3. Data Exposure
When APIs return too much information (like stack traces, internal errors, or sensitive data fields) in response to requests, attackers can use this information to exploit vulnerabilities in the system.
4. Lack of Encryption
APIs that transmit data without encryption, especially over insecure channels (like HTTP), risk exposing sensitive information to man-in-the-middle (MITM) attacks. Attackers can intercept API traffic, steal sensitive data, and tamper with the communication.
5. Rate Limiting and DoS Attacks
APIs without rate limiting are vulnerable to Denial of Service (DoS) attacks, where attackers overwhelm the API with requests, causing it to crash or slow down significantly, affecting the availability of the service.
6. Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF)
These attacks can occur when APIs do not properly sanitize user inputs or fail to use secure tokens to ensure that requests come from legitimate users.
Best Practices for Securing API Endpoints
Securing your API endpoints involves implementing a combination of authentication, encryption, input validation, and monitoring strategies. Below are some essential best practices every developer should follow:
1. Implement Strong Authentication
One of the fundamental steps in securing an API is ensuring that only authenticated users can access it. Authentication verifies the identity of the user or system making the request.
Best Practices for Authentication:
- OAuth 2.0: Use OAuth 2.0, one of the most secure and widely adopted authentication protocols, to issue access tokens that authorize users to interact with your API.
- JWT (JSON Web Tokens): Use JWT for token-based authentication. JWTs are compact, self-contained tokens that verify the user’s identity and contain claims that the API can use for validation.
- API Keys: For simple use cases, API keys can provide authentication. However, they should be used with caution, as they don’t offer the same level of security as OAuth 2.0 or JWT. Ensure that keys are securely stored and regenerated when needed.
Multi-Factor Authentication (MFA):
For highly sensitive APIs, consider implementing multi-factor authentication (MFA), where users must verify their identity with two or more methods (e.g., a password and a one-time code).
2. Use Proper Authorization Mechanisms
Authentication verifies who the user is, but authorization ensures that the user has the correct permissions to access specific resources. Use Role-Based Access Control (RBAC) or Attribute-Based Access Control (ABAC) to enforce fine-grained authorization.
Best Practices for Authorization:
- Least Privilege: Apply the principle of least privilege by limiting access to only those resources that the user needs.
- Scopes and Roles: Use OAuth scopes or custom roles to assign different permission levels based on user roles (e.g., admin, user, guest).
- Endpoint Restrictions: Ensure that each API endpoint has strict access control mechanisms in place. For example, administrative endpoints should only be accessible to users with the correct privileges.
3. Encrypt All Communication (Use HTTPS)
Always encrypt API communication using SSL/TLS (HTTPS). Encryption ensures that sensitive data transmitted between the client and server remains secure, protecting against man-in-the-middle (MITM) attacks.
Best Practices for Encryption:
- HTTPS Only: Ensure that all API traffic is routed through HTTPS and that HTTP traffic is redirected to HTTPS automatically.
- TLS Certificates: Use up-to-date and secure TLS certificates to encrypt communications. Renew certificates regularly.
- Encrypt Sensitive Data at Rest: In addition to securing data in transit, encrypt sensitive data stored in your databases.
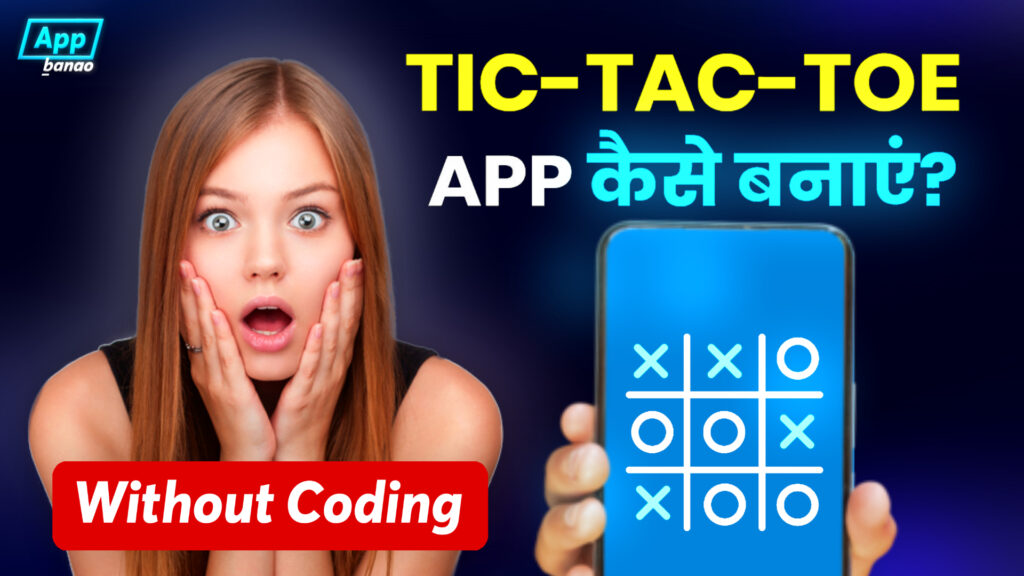
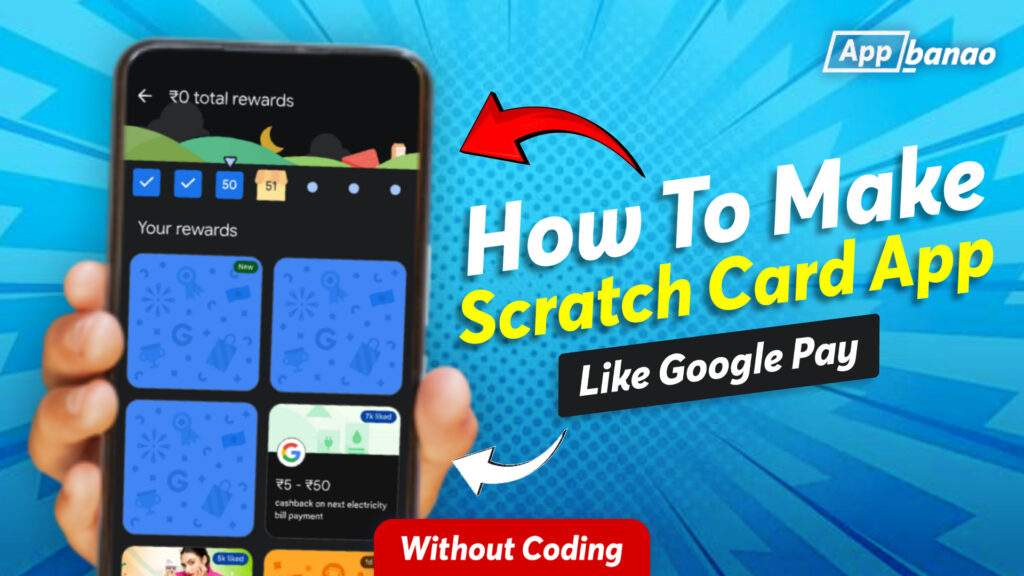
4. Validate and Sanitize Inputs
APIs are highly vulnerable to attacks like SQL injection, XSS, and buffer overflows if they don’t properly validate and sanitize inputs. Always assume that user input is untrusted and can be manipulated.
Best Practices for Input Validation:
- Use Parameterized Queries: Prevent SQL injection by using parameterized queries or prepared statements in your database operations.
- Whitelist Input: Implement input validation by only allowing known, expected input values. For example, validate email addresses using a specific format and reject invalid entries.
- Limit Data Size: Impose limits on the size of data input to prevent buffer overflow attacks or denial-of-service attempts by submitting overly large payloads.
5. Implement Rate Limiting and Throttling
To protect your API from Denial of Service (DoS) attacks or abuse, implement rate limiting and throttling. This ensures that no single user can overwhelm your server with excessive requests.
Best Practices for Rate Limiting:
- Set Request Limits: Define maximum requests per IP address, user, or access token within a specific time frame (e.g., 100 requests per minute).
- Use API Gateway: Leverage an API gateway (e.g., AWS API Gateway, Kong) to manage and enforce rate limits, throttling, and IP filtering.
- Graceful Handling: Implement appropriate error handling (such as returning a
429 Too Many Requests
response) when users exceed their rate limits.
6. Protect Against Cross-Site Request Forgery (CSRF)
CSRF attacks trick users into performing actions they did not intend by submitting requests on their behalf without their knowledge. These attacks can be particularly dangerous if the API is used within web applications.
Best Practices for CSRF Prevention:
- CSRF Tokens: Use secure CSRF tokens that are unique to each session and required for any sensitive API operation (such as modifying data or making purchases).
- Same-Site Cookies: Implement SameSite cookie attributes, which ensure that cookies are not sent along with cross-site requests, mitigating CSRF attacks.
Conclusion
Securing API endpoints is essential in today’s interconnected digital world, where APIs often serve as the backbone of critical applications. By implementing robust security measures, including authentication, encryption, input validation, and continuous monitoring, developers can protect APIs from common vulnerabilities and keep sensitive data safe from attackers.
Taking a proactive approach to API security ensures not only the protection of your app and users but also maintains trust and compliance with industry regulations. Whether you’re building an API for a small project or a large enterprise application, following these best practices will help safeguard your system against potential threats.
Tips for Reducing App Load Time and Memory Usage
- October 17, 2024
- Com 0
In today’s fast-paced digital world, users expect mobile apps to perform flawlessly, load quickly, and run efficiently. An app that…
Strategies to Reduce App Abandonment and Increase Retention
- October 17, 2024
- Com 0
In the highly competitive app marketplace, user retention is one of the most important metrics for measuring success. While acquiring…
Fintech App Development: Ensuring Compliance and Security
- October 16, 2024
- Com 0
The rapid growth of fintech (financial technology) apps has revolutionized the financial services industry. From mobile banking and digital wallets…
The Future of Mobile Apps in the Travel and Tourism Industry
- October 15, 2024
- Com 0
The travel and tourism industry has undergone a significant transformation in recent years, driven largely by advancements in technology. Mobile…
What Every Developer Should Know About Securing API Endpoints
- October 13, 2024
- Com 0
APIs (Application Programming Interfaces) play a critical role in modern application development, enabling communication between client applications and backend services.…
Integrating Cloud Services into Your Mobile App
- October 12, 2024
- Com 0
Cloud services have revolutionized the way modern mobile apps are developed, deployed, and maintained. Integrating cloud services into your mobile…